STL(Standard Template Library,标准模板库)是惠普实验室开发的一系列软件的统称。它是由Alexander Stepanov、Meng Lee和David R Musser在惠普实验室工作时所开发出来的。现在虽说它主要出现在C++中,但在被引入C++之前该技术就已经存在了很长的一段时间。
组成的库来说提供了更好的代码重用机会。在C++标准中,STL被组织为下面的13个头文件:<algorithm>
、<deque>
、<functional>
、<iterator>
、<vector>
、<list>
、<map>
、<memory>
、<numeric>
、<queue>
、<set>
、<stack>
和<utility>
。
STL可分为容器(containers)、迭代器(iterators)、空间配置器(allocator)、配接器(adapters)、算法(algorithms)、仿函数(functors)六个部分。
本文就是主要介绍STL的基本内容。
序列容器
|
|
array
|
|
vector
|
|
示例:
deque
|
|
forward_list
|
|
list
|
|
示例
关联容器
|
|
set
|
|
示例
map
|
|
示例
multiset
|
|
multimap
|
|
无序关联容器
|
|
unordered_set
|
|
unordered_map
|
|
unordered_multiset
|
|
unordered_multimap
|
|
容器适配器
|
|
stack
|
|
示例
queue
|
|
示例
priority_queue
|
|
示例
容器的线程安全性
|
|
最终是一张大表:
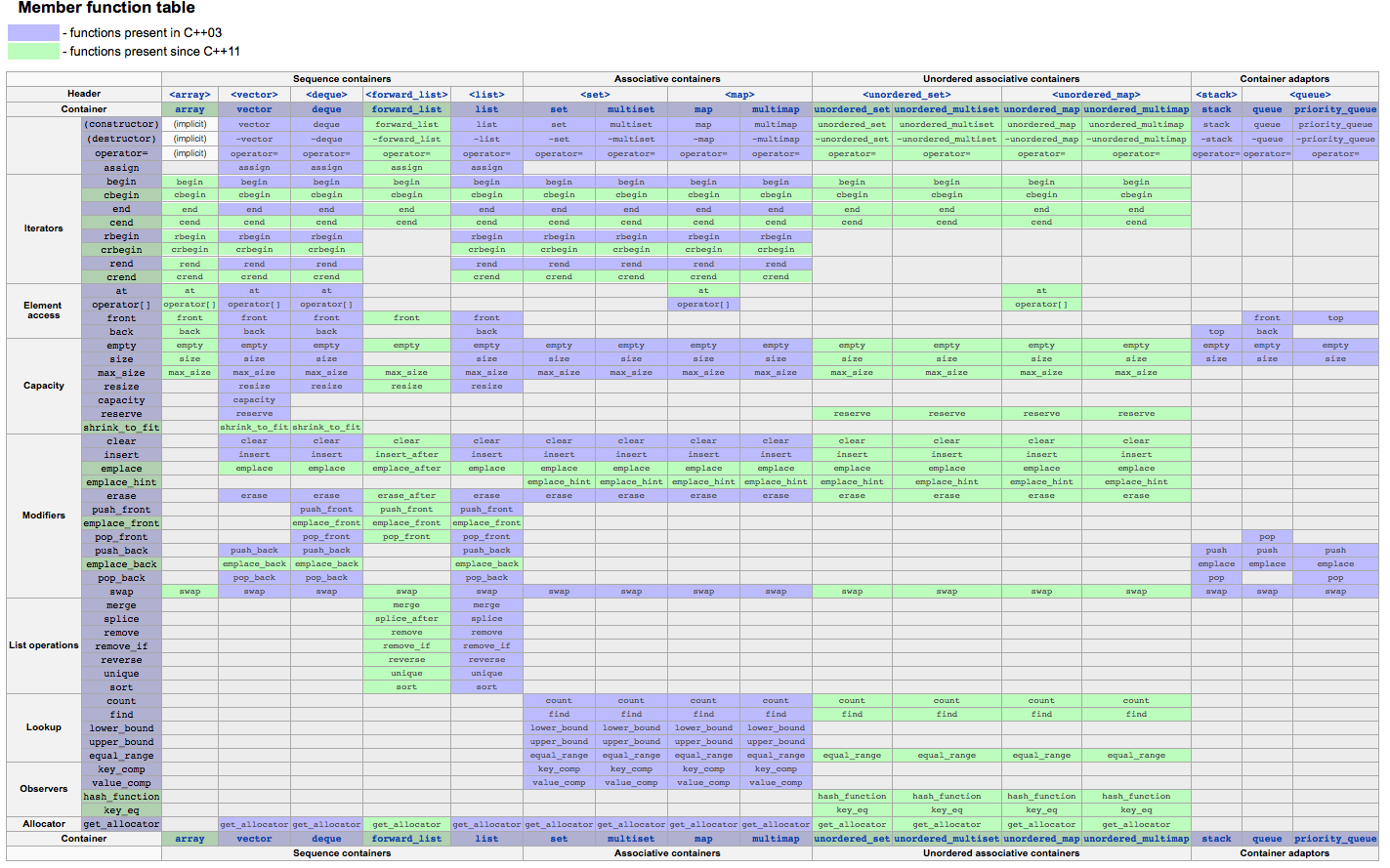